https://www.acmicpc.net/problem/2884
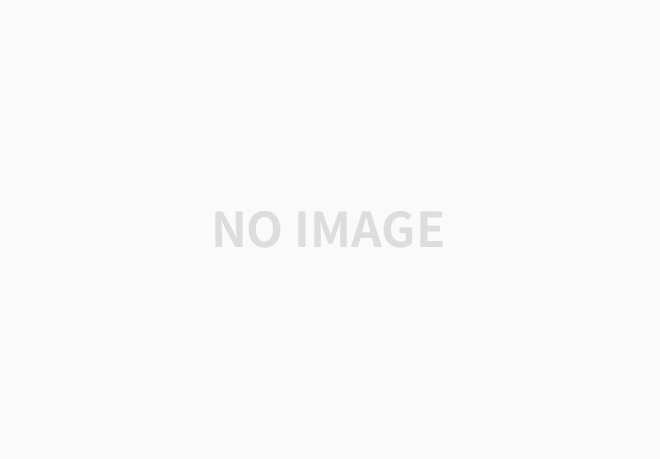
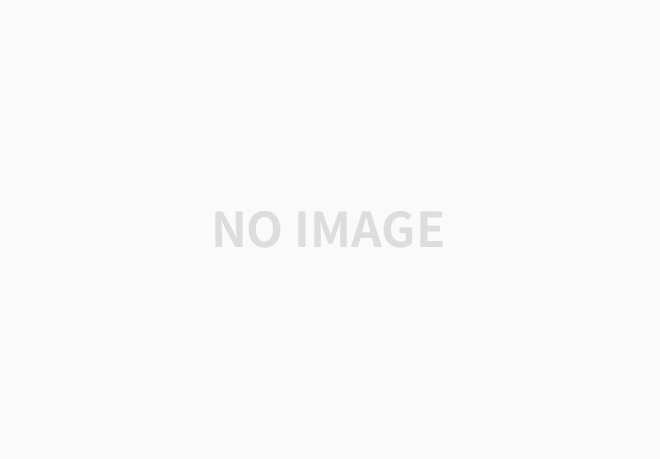
내 답안
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
Scanner s = new Scanner(System.in);
int H = s.nextInt();
int M = s.nextInt();
int H_new = 0;
int M_new = 0;
if (M>=45) {
H_new = H;
M_new = M-45;
System.out.printf("%d %d", H_new, M_new);
}
else {
H_new = H-1;
M_new = 60-(45-M);
if (H_new < 0) {
H_new = 23;
}
System.out.printf("%d %d", H_new, M_new);
}
}
}
[H_new, M_new를 따로 선언하지 않고 푸는 방법]
(+) 출력문을 두 번 적지 않는 방법 또한 찾아내어 적용하였다.
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
Scanner s = new Scanner(System.in);
int H = s.nextInt();
int M = s.nextInt();
if (M>=45) M-=45;
else {
H--;
M = 60-(45-M);
if (H < 0) {
H = 23;
}
}
System.out.printf("%d %d", H, M);
}
}
[분 단위만 사용하여 풀기]
ex) 모든 단위를 분으로 통합 -> 10시 10분 -> 610분
610 - 45 = 565
시간 = 565 / 60 = 몫
분 = 565 % 60 = 나머지
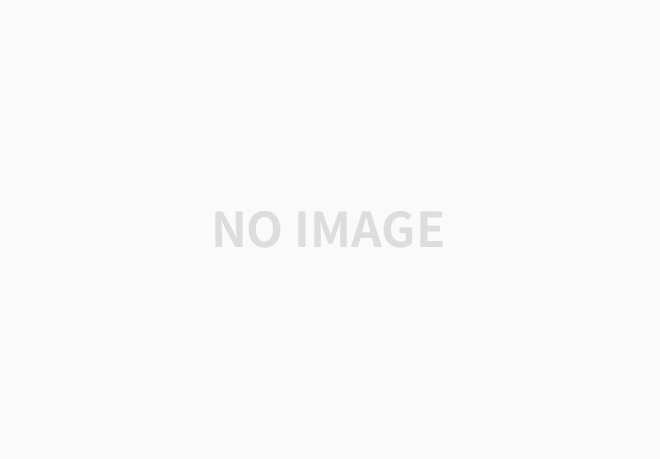
또는 조건문을 이렇게 변경해도 됨
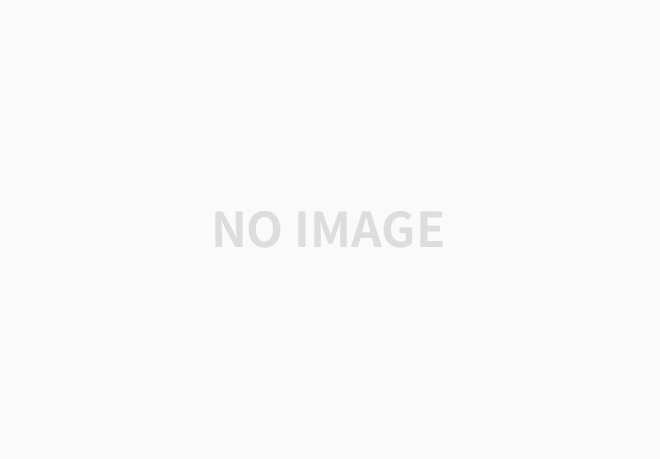
[BufferedReader 사용]
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int h, m, min;
String[] read = br.readLine().split(" ");
h = Integer.parseInt(read[0]);
m = Integer.parseInt(read[1]);
if (m<45 && h==0) h = 24;;
min = h*60+m;
min-=45;
h = (int)(min/60);
m = min%60;
System.out.printf("%d %d", h, m);
}
}
'Coding Test > 백준' 카테고리의 다른 글
[백준 10950번 - java] A + B - 3 (0) | 2021.11.03 |
---|---|
[백준 2739번 - java] 구구단 (0) | 2021.11.03 |
[백준 14681번 - java] 사분면 고르기 (0) | 2021.11.03 |
[백준 1330번 - java] 두 수 비교하기 (0) | 2021.11.03 |
[백준 9498번 - java] 시험 성적 (0) | 2021.11.03 |